Flash is a popular format for browser-based video games on sites like Newsgrounds and Kongregate. Although the Flash format is losing popularity due to the success of mobile applications, many quality games are still made with this technology today. Flash uses ActionScript, an easy-to-learn language that gives you control over objects on the screen. Start from step 1 to learn how to make a simple Flash game.
Steps
Part 1 of 3: Starting the Process

Step 1. Design your game
Before you start coding, it will be helpful to have a rough idea of how your game works. Flash is more suited to simple games, so focus on creating a game that has only a few mechanics that the player needs to worry about. Try to have a genre and some mechanics in mind before you start creating your prototype. The most common Flash games include:
- Infinite Run: In these games the character moves automatically, and the player just needs to jump over obstacles or otherwise interact with the game. The player will typically only have one or two control options.
- Beat 'em up: These games are typically scrolling and the player will have to defeat enemies to progress. The character often has several moves at his disposal to defeat enemies.
- Puzzles: These games require the player to solve puzzles to pass each level. These can be games that require you to create combinations of three objects, such as Bejeweled or more complex puzzles commonly found in adventure games.
- RPG: These games focus on character development and progression, and the player will have to move through multiple environments while facing a variety of enemies. Combat mechanics vary a lot between RPGs, but many of them are turn-based. RPGs can be much more difficult to program than simple action games.
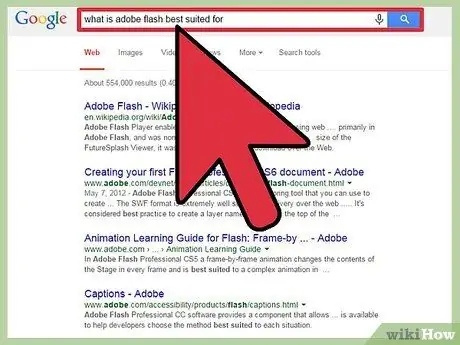
Step 2. Learn the best aspects of Flash
Flash is suitable for 2D games. It is possible to create 3D games in Flash, but advanced techniques and significant knowledge of the language are required. Almost all successful Flash games are 2D.
Flash games are best suited for short gaming sessions. This is because most people who play Flash video games do so when they have little free time, such as during a break, and this means that sessions typically last 15 minutes or less

Step 3. Familiarize yourself with the ActionScript3 (AS3) language
Flash games are programmed in AS3, and you will need to understand the basic operation of this language to be able to successfully create a game. You can create a simple game with a rudimentary level of understanding how to program in AS3.
On Amazon and in bookstores you can find many ActionScript texts, as well as many guides and examples on the internet

Step 4. Download Flash Professional
This program is paid, but it is the best way to quickly create Flash programs. Other options are available, including some open-source ones, but they often have compatibility issues or take longer to complete the same tasks.
Flash Professional is the only program you will need to start making games
Part 2 of 3: Writing a Simple Game

Step 1. Get to know the basic building blocks of the AS3 code
When you are creating a simple game, you will use many different code structures. There are three main parts of any AS3 code:
-
Variables - this is where your data is stored. The data can be numbers, words (strings), objects and more. Variables are defined by var code and must be one word.
var Player Health: Number = 100; // "var" indicates that you are defining a variable. // "healthPlayer" is the name of the variable. // "Number" is the data type. // "100" is the value assigned to the variable. // All actionscript lines end with ";"
-
Event Handlers - Event handlers look for specific events and when they happen they communicate it to the rest of the program. They are essential for handling player controls and for repeating code. Event handlers can typically call functions.
addEventListener (MouseEvent. CLICK, fendenteSpada); // "addEventListener ()" defines the event handler. // "MouseEvent" is the category of the input that is expected. // ". CLICK" is the specific event in the MouseEvent category. // "fendenteSpada" is the function that is called when the event occurs.
-
Functions - the sections of code assigned to a keyword that can be called later. Functions handle most of the game's programming, and complex games can have hundreds of functions, while simpler ones only have a few. They can be written in any order, because they only work when called.
function fendenteSpada (e: MouseEvent): void; {// Here you will have to enter the code} // "function" is the keyword that appears at the beginning of each function. // "fendenteSpada" is the name of the function. // "e: MouseEvent" is an additional parameter, which shows that the function // is being called from an event handler. // ": void" is the value returned by the function. If no value // is to be returned, use: void.
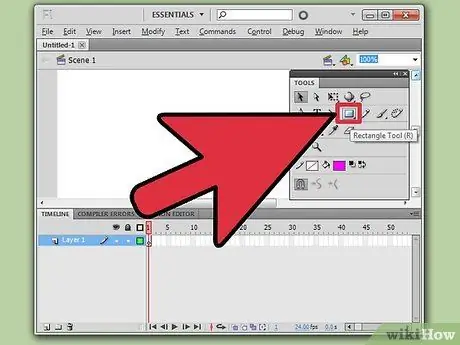
Step 2. Create an object
ActionScript is used to affect objects in Flash. To create a game, you will need to create objects that the player can interact with. According to the guides you are reading, the objects could be called sprites, actors or movie clips. For this simple game, you will create a rectangle.
- Open Flash Professional if you haven't already. Create a new ActionScript 3 project.
- Click on the Rectangle drawing tool from the Tools panel. This panel can be in different locations depending on the Flash Professional configuration. Draw a rectangle in your Scene window.
- Select the rectangle using the Selection tool.

Step 3. Assign properties to the object
After selecting your newly created rectangle, open the Edit menu and select "Convert to Symbol". You can also press F8 as a shortcut. In the "Convert to Symbol" window, give the object an easy to recognize name, such as "enemy".
- Find the Properties window. At the top of the window, you will see an empty text field called "Instance Name" when you move your mouse over it. Type the same name you entered when converting to symbol ("enemy"). This will create a unique name with which you can interact with the AS3 code.
- Each "instance" is a separate object that can be influenced by code. You can copy the already created instance multiple times by clicking on the Library tab and dragging the instance onto the scene. Each time you add one, the name will be changed to indicate that it is a separate item ("enemy", "enemy1", "enemy2", etc.).
- When referencing objects in your code, you simply need to use the instance name, in this case "enemy".

Step 4. Learn how to change the properties of an instance
Once an instance is created, you can modify its properties with AS3. This way you can move the object on the screen, resize it, and so on. You can change the properties by typing the instance, followed by a period ".", Followed by the property and finally the value:
- enemy.x = 150; This changes the position of the enemy object on the X axis.
- enemy.y = 150; This command changes the position of the enemy object on the Y axis. The Y axis is calculated from the top of the scene.
- enemy.rotation = 45; Rotate the enemy object 45 degrees clockwise.
- enemy.scaleX = 3; Stretch the width of the object by a factor of 3. A number (-) will invert the object
- enemy.scaleY = 0.5; Reduces the height of the object in half.

Step 5. Examine the trace () command
This command returns the current value of the specified objects, and is useful for figuring out if everything is done correctly. You may not include the trace command in the final code, but it is useful when debugging.

Step 6. Create a simple game with the information provided so far
Now that you have a basic understanding of the main functions, you can create a game where an enemy changes its size every time you click on it, until its health is depleted.
var health Enemy: Number = 100; // set the enemy's health to 100. var attackPlayer: Number = 10; // set the player's attack power when he clicks. enemy.addEventListener (MouseEvent. CLICK, attacks Enemy); // By adding this function directly to the enemy object, // the function will only be called when the object itself is clicked // and not anywhere else on the screen. setposition Enemy (); // This command calls the following function to place the enemy // on the screen. This happens when the game starts. function setpositionEnemy (): void {enemy.x = 200; // place the enemy 200 pixels from the left of the enemy screen.y = 150; // place the enemy 150 pixels from the top of the enemy screen.rotation = 45; // rotate enemy 45 ° clockwise trace ("enemy's x-value is", enemy.x, "and enemy's y-value is", enemy.y); // Show the enemy's current position for errors} function attackEnemy (e: MouseEvent): void // This command creates the attack function for when the enemy is clicked {enemy health = enemy health - player attack; // Subtract the attack value from the health value // resulting in the new health value. enemyy.scaleX = enemy health / 100; // Change the width of the enemy based on their health. // The value is divided by 100 to make it a decimal. enemy.scaleY = health Enemy / 100; // Modify the enemy's height based on their health. trace ("The enemy has", health Enemy); // Returns enemy health}

Step 7. Try the game
When you have created the code, you can try your new game. Click on the Control menu and select Test Movie. The game will start, and you can click on the enemy object to change its size. In the Output window you will see the results of the trace command.
Part 3 of 3: Learning the Advanced Techniques

Step 1. Learn how packages work
ActionScript is based on Java, and uses a very similar package system. Packages allow you to store variables, constants, functions, and other information in separate files, and then import these files into your program. This is especially useful if you want to use a package developed by someone else that will simplify the creation of your game.

Step 2. Create the project folders
If you are creating a game with a lot of images and sound clips, you should create a folder structure for your game. This will allow you to easily archive the different items, as well as store the different packages to call.
- Create a base folder for your project. In the base folder, you should create an "img" folder for all graphics components, a "snd" folder for all sounds, and a "src" folder for all game packages and code.
- Create a "Game" folder in the "src" folder to store your Constants files.
- This particular structure is not necessary, but it allows you to easily sort your work and your materials, especially in cases of larger projects. For the simple game described above, you don't need to create any folders.

Step 3. Add sound to your game
A game without sound or music will quickly bore the player. You can add sounds to objects in Flash using the Layers tool.

Step 4. Create a Constants file
If your game has a lot of values that don't change over the course of a game, you can create a Constants file to keep them all in one place for easy recall. Constants can include values such as gravity, player speed, and other values that you will need to call repeatedly.
-
If you create a Constants file, you will need to place it in a folder of your project and then import it as a package. For example, let's say you created a Constants.as file and saved it in the Game folder. To import it you will need to use the following code:
package {import Game. *; }

Step 5. Study other people's games
While many developers do not disclose the code of their games, there are many guides and other open projects that will allow you to visualize the code and how it interacts with game objects. This is a great way to learn advanced techniques that can make your game stand out.