The "C" programming language is one of the oldest - it was developed in the 1970s - but it is still very powerful due to its low-level structure. Learning C is a great way to prepare for more complex languages, and the notions you will learn will be useful for almost any programming language. To learn how to start programming in C, read on.
Steps
Part 1 of 6: Preparation
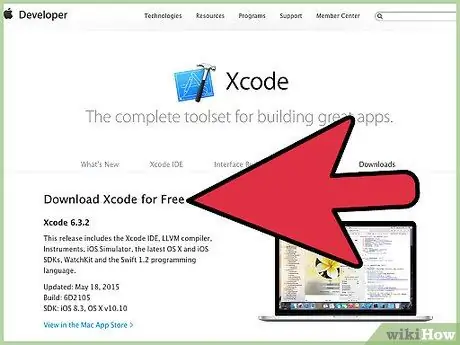
Step 1. Download and install a compiler
The C code must be compiled by a program that interprets the signal code that the machine can understand. Compilers are usually free and you can find several for different operating systems.
- On Windows, try Microsoft Visual Studio Express or MinGW.
- For Mac, XCode is one of the best C compilers.
- For Linux, gcc is one of the most used options.

Step 2. Learn the basics
C is one of the older programming languages, and it can be very powerful. It was designed for Unix operating systems, but has been adapted and expanded for almost all operating systems. The modern version of C is C ++.
C is basically understood by functions, and in these functions you will be able to use variables, conditional statements and loops to hold and manipulate data

Step 3. Review some base codes
Look at the following program (very simple) to get an idea of how some aspects of the language work and to become familiar with how the programs work.
#include int main () {printf ("Hello, World! / n"); getchar (); return 0; }
- The #include command is placed before the program starts and loads the libraries that contain the functions you need. In this example, stdio.h allows us to use the printf () and getchar () functions.
- The int main () command tells the compiler that the program is executing the function called "main" and that it will return an integer when it is done. All C programs execute a "main" function.
- The symbols "{" and "}" indicate that everything inside them is part of a function. In this case, they denote that everything inside is part of the "main" function.
- The printf () function displays the contents of the bracket on the user's screen. The quotation marks ensure that the string inside is printed literally. The sequence / n tells the compiler to move the cursor to the next line.
- The; denotes the end of a line. Most lines of code in C must end with a semicolon.
- The getchar () command tells the compiler to wait for a user to press a button before moving forward. This is useful, because many compilers run the program and immediately close the window. In this case, the program will not close until a key is pressed.
- The return 0 command indicates the end of the function. Notice how the "main" function is an int function. This means that it will have to return an integer at the end of the program. A "0" indicates that the program ran successfully; any other number will mean that the program has encountered an error.

Step 4. Try compiling the program
Type the code in a text editor and save it as a "*.c" file. Compile it with your compiler, typically by clicking the Build or Run button.

Step 5. Always comment on your code
Comments are non-compiled parts of the code, which allow you to explain what is happening. This is useful for remembering what your code is for, and for helping other developers who may be using your code.
- To comment in C insert / * at the beginning of the comment and * / at the end.
- Comment on all but the simplest parts of the code.
- You can use comments to quickly remove parts of the code without deleting them. Simply enclose the code to exclude with comment tags and then compile the program. If you want to add the code again, remove the tags.
Part 2 of 6: Using Variables

Step 1. Understand the function of variables
Variables allow you to store data, obtained from program computations or with user input. Variables must be defined before they can be used, and there are several types to choose from.
Some of the more common variables include int, char, and float. Each is used to store a different type of data

Step 2. Learn how to declare variables
Variables must be established, or "declared", before they can be used by the program. You can declare a variable by entering the data type followed by the variable name. For example, the following are all valid variable declarations:
float x; char name; int a, b, c, d;
- Note that you can declare multiple variables on the same line as long as they are of the same type. Simply separate the variable names with commas.
- Like many lines of C, each variable declaration line must end with a semicolon.

Step 3. Learn when to declare variables
You must declare the variables at the beginning of each block of code (the parts included in the brackets {}). If you declare a variable later in the block, the program will not work correctly.
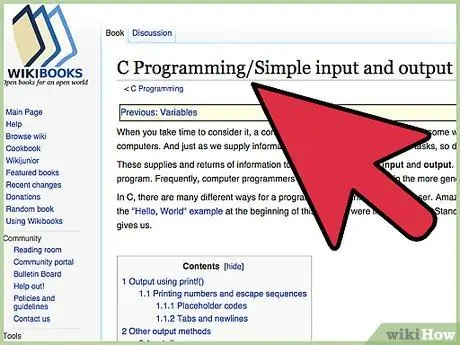
Step 4. Use variables to store user inputs
Now that you know the basics of how variables work, you can write a simple program that stores user input. You will use another function in the program, called scanf. This works searches the supplied inputs for specific values.
#include int main () {int x; printf ("Enter a number:"); scanf ("% d", & x); printf ("You entered% d", x); getchar (); return 0; }
- The string "% d" tells scanf to look for integers in the user input.
- The & before the variable x tells scanf where to find the variable to modify it and stores the integer in the variable.
- The final printf command returns the entered integer to the user.

Step 5. Manipulate your variables
You can use mathematical expressions to manipulate the data you have stored in your variables. The most important distinction to remember for math expressions is that a single = assigns a value to the variable, while == compares the values from both sides to make sure they are equal.
x = 3 * 4; / * assigns "x" to 3 * 4, or 12 * / x = x + 3; / * adds 3 to the original value of "x", and assigns the new value as a variable * / x == 15; / * checks that "x" is equal to 15 * / x <10; / * check if the value of "x" is less than 10 * /
Part 3 of 6: Using Conditional Statements

Step 1. Understand the basics of conditional statements
These claims are at the heart of many programs. These are statements that can be true (TRUE) or false (FALSE) and tell the program how to act according to the result. The simplest statement is if.
TRUE and FALSE work differently than you might imagine on C. TRUE statements always end by equating to a non-zero number. When you perform a comparison, if the result is TRUE, the function will return the value "1". If the result is FALSE, the function will return a "0". Understanding this concept will help you understand how IF statements are processed

Step 2. Learn the basic conditional operators
Conditional statements are based on the use of mathematical operators that compare values. The following list contains the most used conditional operators.
/ * greater than * / </ * less than * /> = / * greater than equal * / <= / * less than equal * / == / * equal to * /! = / * not equal to * /
10> 5 TRUE 6 <15 TRUE 8> = 8 TRUE 4 <= 8 TRUE 3 == 3 TRUE 4! = 5 TRUE

Step 3. Write a simple IF statement
You can use IF statements to determine what the program should do after evaluating the statement. You can combine them with other conditional statements later to create powerful multiple options, but for now, write a simple one to get used to.
#include int main () {if (3 <5) printf ("3 is less than 5"); getchar (); }

Step 4. Use ELSE / ELSE IF statements to expand your terms
You can expand IF statements by using ELSE and ELSE IF to handle the different results. ELSE statements are performed if the IF statement is FALSE. ELSE IF statements allow you to include multiple IF statements in a single block of code to handle various cases. Read the sample program below to see their interaction.
#include int main () {int age; printf ("Enter your current age please:"); scanf ("% d", $ age); if (age <= 12) {printf ("You're just a kid! / n"); } else if (age <20) {printf ("Being a teenager is the best! / n"); } else if (age <40) {printf ("You are still young in spirit! / n"); } else {printf ("As you get older you get wiser. / n"); } return 0; }
The program receives user input and parses it with the IF statement. If the number satisfies the first statement, the program will return the first printf. If it does not satisfy the first statement, all ELSE IF statements will be considered until the one satisfied is found. If none of the statements are satisfied, the ELSE statement will be executed at the end of the block
Part 4 of 6: Learning to Use Loops

Step 1. Understand how loops work
Loops are one of the most important aspects of programming, because they allow you to repeat blocks of code until specific conditions are met. This greatly simplifies the implementation of repeated actions, and allows you to not have to rewrite new conditional statements every time you want to make something happen.
There are three main types of loops: FOR, WHILE, and DO… WHILE

Step 2. Use a FOR loop
This is the most common and useful type of loop. It will continue to execute the function until the conditions of the FOR loop are met. FOR loops require three conditions: the initialization of the variable, the condition to satisfy, and the method of updating the variable. If you don't need these conditions, you'll still have to leave a blank space with a semicolon, or the loop will run non-stop.
#include int main () {int y; for (y = 0; y <15; y ++;) {printf ("% d / n", y); } getchar (); }
In the previous program, y is set to 0, and the loop continues until the value of y is less than 15. Each time the value of y is printed, 1 is added to the value of y and the loop is repeated. When y = 15, the loop will stop

Step 3. Use a WHILE loop
WHILE loops are simpler than FOR loops. They only have one condition, and the loop runs as long as that condition is true. You don't need to initialize or update the variable, although you can do that in the main body of the loop.
#include int main () {int y; while (y <= 15) {printf ("% d / n", y); y ++; } getchar (); }
The y ++ command adds 1 to the y variable each time the loop is executed. When y reaches 16 (remember, the loop runs until y is less than 15), the loop stops

Step 4. Use a DO loop
.. WHILE. This loop is very useful for loops you want to make sure they are played at least once. In FOR and WHILE loops, the condition is checked at the beginning of the loop, which means that it may not be met and end the loop right away. DO… WHILE loops check the conditions at the end of the loop, ensuring that the loop is executed at least once.
#include int main () {int y; y = 5; do {printf ("This loop is running! / n"); } while (y! = 5); getchar (); }
- This loop will show the message even if the condition is FALSE. The variable y is set to 5 and the WHILE loop has the condition that y is different from 5, so the loop will end. The message was already printed, because the condition was not checked before the end.
- The WHILE loop in a DO… WHILE series must end with a semicolon. This is the only case where a loop is closed by a semicolon.
Part 5 of 6: Using the Functions

Step 1. Understand the basics of functions
Functions are blocks of code that can be called elsewhere in the program. They greatly simplify code repetition, and help in reading and editing the program. The functions can include all the techniques described above, as well as other functions.
- The main () line at the beginning of all previous examples is a function, as is getchar ()
- Functions are essential for creating efficient and easy-to-read code. Use the functions well to create a clear and well-written program.

Step 2. Start with a description
The best way to create functions is to start with a description of what you want to achieve before you start coding. The basic syntax of functions is "return_type name (argument1, argument2, etc.);". For example, to create a function that adds two numbers:
int sum (int x, int y);
This will create a function that sums two integers (x and Template: kbdr) and then returns the sum as an integer
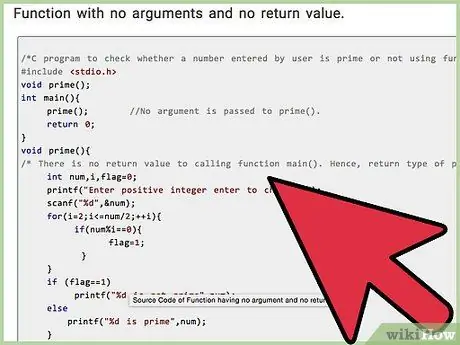
Step 3. Add the function to a program
You can use the description to create a program that takes two user-entered integers and adds them together. The program will define the operation of the "add" function and use it to manipulate the numbers entered.
#include int sum (int x, int y); int main () {int x; int y; printf ("Type two numbers to add:"); scanf ("% d", & x); scanf ("% d", & y); printf ("The sum of the numbers is% d / n" sum (x, y)); getchar (); } int sum (int x, int y) {return x + y; }
- Note that the description is still at the beginning of the program. This will tell the compiler what to expect when the function is called and what the result will be. This is only necessary if you don't want to define the function later in the program. You could define sum () before the main () function and the result would be the same even without the description.
- The true functionality of the function is defined at the end of the program. The main () function collects the integers entered by the user and then passes them to the sum () function for manipulation. The sum () function will return the results to the main () function
- Now that the add () function has been defined, it can be called anywhere in the program.
Part 6 of 6: Keep Learning

Step 1. Find some books on C programming
This article teaches the basics, but just scratches the surface of C programming and all the notions associated with it. A good reference manual will help you troubleshoot and save you a lot of headaches.

Step 2. Join a community
There are many communities, online or real, dedicated to programming and all existing languages. Find C programmers like you to exchange ideas and code with, and you'll learn a lot from them.
Participate in programming marathons (hack-a-thon) These are events where groups and people have to invent programs and solutions within a time limit, and they stimulate creativity a lot. You can meet many good programmers this way, and you will find hack-a-thons all over the world

Step 3. Take courses
You won't have to go back to school and get a Computer Science degree, but taking a few courses can help you a lot to learn. Nothing beats the direct help of people skilled in the use of language. You will often find courses in universities, and in some cases you will be able to participate without registering.

Step 4. Consider learning the C ++ language
Once you've learned about C, it won't hurt to start considering C ++. This is the modern version of C, which allows for much more flexibility. C ++ is designed to handle objects, and knowing this language allows you to create powerful programs for almost any operating system.
Advice
- Always add comments to your schedules. This will not only help those dealing with your source code, but will also help you remember what you are writing and why. You may know what to do by the time you write the code, but after two or three months, remembering won't be that easy.
- When you find a syntax error while compiling, if you can't move forward, do a Google search (or another search engine) with the error you received. Someone has probably already had the same problem as you and posted a solution.
- Your source code must have the extension *.c, so that your compiler can understand that it is a C source file.