This article is intended to give new MATLAB users a basic introduction to graphing data. It's not meant to cover every detail of graphing in MATLAB, but it should cover enough to get you started. This introduction does not require any previous programming experience and will explain any common programming construct used within.
Steps

Step 1. You need to know a few things about MATLAB
- Comma operator: if a command is followed by a ';' the output will not be printed on the screen. This is trivial when the output is a short assignment, such as y = 1, but becomes problematic if a large array is created. Also, whenever you want output, such as a graph, the semicolon must be omitted.
- Clear command: There are some useful command window commands. Typing "clear" in the command window after the >> prompt will clear all current variables, which can help if you see an unusual exit. Also, you can type "clear" followed by a variable name to clear only the data for that specific variable.
- Types of Variables: The only type of variable in MATLAB is an array or vector. This means that variables are stored as lists of numbers, with the simplest list containing only a number. In the case of MATLAB, the size of the array may not be specified when the variable is created. To set a variable to a single number, you simply type something like z = 1. If you then want to add something to z, you can simply write z [2] = 3. You can then refer to the number stored anywhere in the vector by typing z , where "i" is the i-th position of the vector. So if you want to get the value 3 from example z, just type z [2].
- Loops or Loops: Loops are used when you want to perform an action multiple times. There are two common types of loops in MATLAB, the for loop and the while loop. Both can usually be used interchangeably, but it is easier to create an infinite loop with the while than with the for. You can tell if you have dropped an infinite loop when the computer stops and gives nothing out except what is inside the loop.
- For loop: These loops in MATLAB take the form of: for i = 1: n / do something / end (slash indicates a line break). This cycle means "do something" n times. So if it prints "Hello" every time the instruction enters the loop and n equals 5, then it should print "Hello" five times.
- While loop: while loops in MATLAB take the form of: while statement is true / do something / end. This cycle means "do something", while the statement is true. Usually the "do something" contains a part that makes the statement false. To do a while loop similar to the previous for loop, you can type while i <= n / do something / end.
- Nested Loops: A nested loop occurs when one loop is inside another. It could be: for i = 1: 5 / for j = 1: 5 / do something / end / end. This would have to "do something" 5 times for j, then increment i, "do something" 5 times for j, increment i and so on.
- For more information on any part of this tutorial or MATLAB in general, visit the MATLAB documentation.

Step 2. Open MATLAB
The window should look like this:

Step 3. Create a new Function file
You don't have to complete this step if you are simply drawing a basic function like y = sin (x). If this is the case, move on to 'step 4'. To create a function file, simply select New from the File menu, then select Function from the drop-down menu. You should get a window similar to the following. This is the window where you should write your functions.

Step 4. Set Your File Function
Delete the [output args] portion and the "=" sign. These are only needed if you want an output value, which is not needed for the graphical representation. Change the "Untitled" part to the name you want the function to have. Enter a variable name instead of "input args". From here on we will use "n" as the input argument. You will use this variable to tell the program how many data points you want. The code should look like this: You can delete the parts after the% marks or leave them - it's up to you, as anything following the '%' symbol is considered a comment and will be ignored by the computer when the function is executed.

Step 5. Set up your details
This step can be achieved in several ways depending on the type of data you want to represent. If you want to plot a simple function like y = sin (x), use the simple method. If you need to plot a data series with increasing x, for example (1, y1), (2, y2),… (n, yn), but you want to use a variable number of points, then use the vector method. If, on the other hand, you want to generate a bullet list with 3 variables instead of 2, the matrix method will be more useful.
- Simple method: decide which range of x you want to use for independent variables and by how much you want to increment it each time. For example, ">> x = 0: (pi / 100): (2 * pi);" will set x between 0 and 2 * Pigreco with intervals of Pi / 100. The middle part is optional and by default it is set in intervals of 1. For example, x = 1:10 will assign the numbers 1, 2, 3,… 10 to x. Type the function on the command line in the command window. It will look like ">> y = sin (x);"
- 'Vector method': set up a For loop to insert values into a vector. Vector assignments in MATLAB follow the form x (i) = 2, where "i" is greater than zero. You can also refer to parts of the vector that already have a value, such as x (3) = x (2) + x (1). See the Loop section for hints. Keep in mind: n is the number you will use to determine the number of data points. Eg:
- Matrix method: set two nested loops, i.e. one loop within another. The first loop should check the x values, while the second loop should check the y values. Pressing Tab before the second loop can help you keep track of which loop is active at that point. Type your equation inside the second loop, which will be the value given to z. Matrix assignments follow the form x (i, j) = 4, where "i" and "j" are two numbers greater than zero. Remember: n is the number you will use to determine the number of data points. Eg:

Step 6. Now set up your chart
-
Simple method and vector method: Write plot (x) after your For loop if you used the vector method. If you used the simple method, type plot (x, y) and press Enter, then go to step 8. The general form of the Plot function is plot (x, y) where x and y are lists of numbers. Typing plot (z) will plot the values of z for 1, 2, 3, 4, 5, etc… You can choose the color, linetype and shape of the points by adding a string to the arguments of the Plot function. It could be plot (x, y, 'r-p'). In this case, the 'r' would make the red line, the '-' would make a straight line between the points and the 'p' would make the points look like stars. Formatting must be delimited with apostrophes.
- Matrix method: write mesh (x) after your nested loops. Make sure you don't add a semicolon after the mesh or plot declarations.
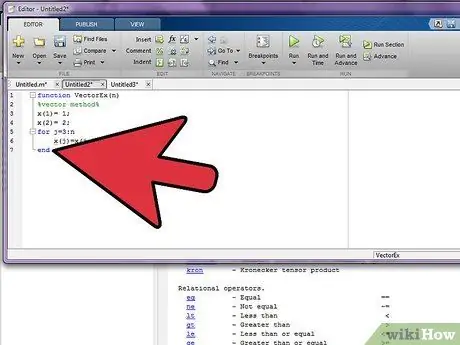
Step 7. Make sure the last line in the function file is "end" and save the file
Skip this step if you used the simple method. Examples of final code for vector and matrix methods are as follows.
- Vector method:
- Matrix method:

Step 8. Run the function
This is done by typing name (n) in the command window, where "name" is the name of the function and "n" is the number of dots you want. Example: ">> FibGraph (8)".
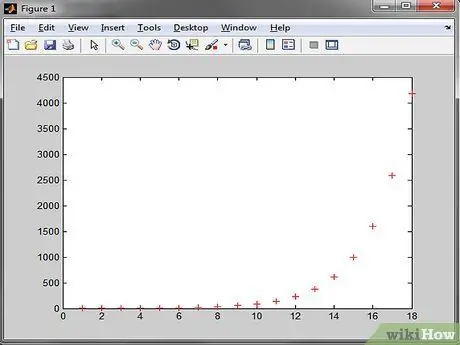
Step 9. Show the results
A window should open with the graph.
- Vector method:
- Matrix method: