Have you ever wanted to program in C ++? The best way to learn is to read other sources. Take a look at some simple C ++ code to learn the structure of a C ++ program and possibly create your own program.
Steps

Step 1. Get a compiler and / or IDE
Three good products are GCC, or if you use Windows, Visual Studio Express Edition or Dev-C ++.
Step 2. Some example programs (copy and paste the following code into a text or code editor):
A simple program was created by Bjarne Stroustrup (the creator of C ++) to control his own compiler:
#include #include using namespace std; int main () {string s; cout << "jhun / n"; cin >> s; cout << "Hello," << s << '\ n'; return 0; // this instruction is not needed}

#include using namespace std; int main () {int no1, no2, sum; cout << "\ nPlease enter the first number ="; cin >> no1; cout << "\ nPlease enter the second number ="; cin >> no2; sum = no1 + no2; cout << "\ nThe sum of" << no1 << "and" << no2 << "=" << sum '\ n'; return 0; }

#include int main () {int sum = 0, value; std:: cout << "Please enter the numbers:" << std:: endl; while (std:: cin >> value) sum * = value; std:: cout << "Sum is:" << sum << std:: endl; return 0; }

#include int main () {int v1, v2, range; std:: cout << "Please enter two numbers << std:: endl; std:: cin >> v1 >> v2; if (v1 <= v2) {range = v2-v1;} else {range = v1-v2;} std:: cout << "range =" << range << std:: endl; return 0;}

#include using namespace std; int main () {int value, pow, result = 1; cout << "Please enter the operand:" << endl; cin >> value; cout << "Please enter the exponent:" << endl; cin >> pow; for (int cnt = 0; cnt! = pow; cnt ++) result * = value; cout << value << "The power of" << pow << "is:" << result << endl; return 0; }

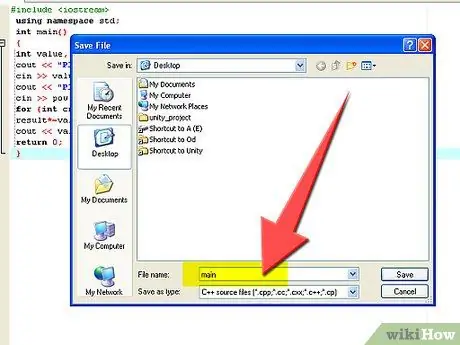
Step 3. Save this file in.cpp format with a name of your choice (yourname.cpp)
Don't get confused by the various c ++ file extensions, just choose one (like *.cc, *.cxx, *.c ++, *.co).
SUGGESTION: In the "Save as" window, select "Save as type"> "All files"
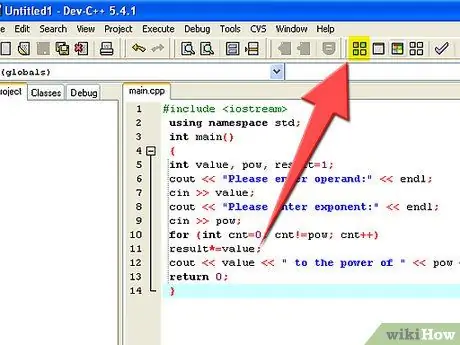
Step 4. Compile the file
For Linux and GCC users, use the g ++ sum.cpp command. On Windows, you can use any C ++ compiler, such as MS Visual C ++, Dev C ++, or any other compiler.

Step 5. Run the program - On Linux use this command:
./a.out (a.out is an executable file produced by the compiler after compiling the program).
Advice
- cin.ignore () prevents the program from closing suddenly, also closing the command line window! To close the program, you will have to press any key.
- Feel free to experiment!
- Use // to comment out the code.
- For more details on C ++ programming, visit cplusplus.com
- Learn to code with ISO standards.
Warnings
- Avoid Dev C ++, because it has numerous bugs, has an outdated compiler, and hasn't been updated since 2005.
- If you try to insert alphabetic values in "int" variables the program will crash. Since you have not written a function to correct the error, the program will not be able to convert the values. Better to use a "string" variable or a more suitable variable depending on the use of the program.
- Never use outdated code.