This article explains how to compile a program written in C using the GNU Compiler (GCC) compiler for Linux or the Minimalist Gnu (MinGW) compiler for Windows.
Steps
Method 1 of 2: Use the GCC Compiler for Linux

Step 1. Open the "Terminal" window on your Linux computer
Normally, it has a black icon with a white command prompt inside. You can find it in the "Applications" menu.
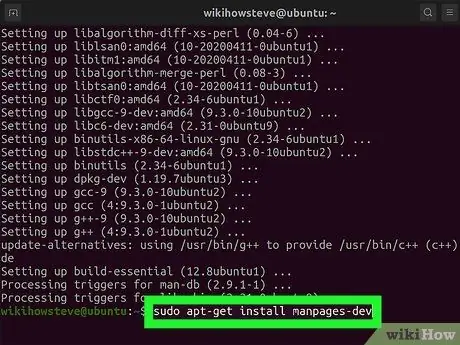
Step 2. Install the GCC compiler
If you haven't already, you can use the "Terminal" window to install the GCC compiler on Ubuntu and Debian systems. For all other Linux distributions you will need to consult their documentation to find out how to get the correct package:
- Type the command sudo apt update and press "Enter" to update the package list.
- Type the command sudo apt install build-essential and press the "Enter" key to install all essential packages, including the one for the GCC, G ++ and Make compilers.
- Type the command sudo apt-get install manpages-dev and press the "Enter" key to install the Linux instruction manual.
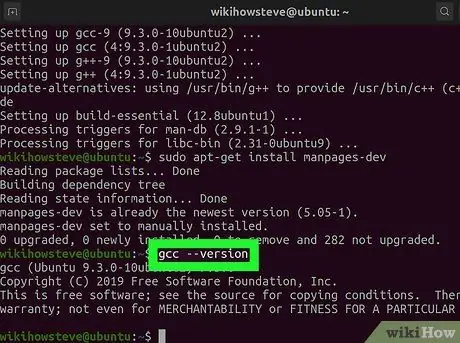
Step 3. Type the command gcc --version and press the Enter key
This step is to verify that the GCC compiler has been installed correctly and, at the same time, to view the version number. If the command is not found, the GCC compiler has not been installed.
If you need to compile a program written in C ++, you will need to use the "g ++" command instead of the "gcc" command

Step 4. Go to the folder where the file containing the source code to compile is stored
Use the cd command inside the "Terminal" window to access the directory you need. For example, if the program file to be compiled is stored in the "Documents" folder, you will need to type the following command cd / home / [username] / Documents (in Ubuntu). Alternatively, you can use the following cd ~ / Documents command within the "Terminal" window.
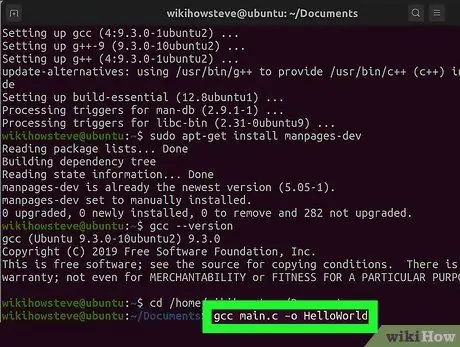
Step 5. Type the command gcc [program_name].c -o [executable_filename] and press the Enter key
Replace the "[program_name].c" parameter with the name of the file that contains the source code to be compiled and the "[executable_filename]" parameter with the name you want to assign to the compiled program. The program will compile immediately.
- If errors are found and you want to know more about them, use the command gcc -Wall -o errorlog [program_name].c. After compiling, view the contents of the "errorlog" log file created in the current working directory using the cat errorlog command.
- To compile a program using multiple source codes, use the command gcc -o outputfile file1.c file2.c file3.c.
- To compile multiple programs at the same time and featuring multiple source files, use the command gcc -c file1.c file2.c file3.c.

Step 6. Run the program you just compiled
Type the command./ [executable_filename] replacing the parameter "[executable_filename]" with the name you assigned to the program's executable file.
Method 2 of 2: Use the MinGW Compiler for Windows

Step 1. Download the GNU Minimalist Compiler for Windows (MinGW)
This is a version of the GCC compiler for Windows systems which is very simple to install. Follow these instructions to download MinGW to your computer:
- Visit the website https://sourceforge.net/projects/mingw/ using your computer browser;
- Click on the green button Download;
- Wait for the installation file to download automatically.


Step 2. Install MinGW
Follow these instructions:
- Double-click the file mingw-get-setup.exe present in the "Download" folder or in the browser window;
- Click on the button Install;
-
Click on the button Continue.
MinGW developers recommend using the default installation folder (C: / MinGW). However, if you need to change it, do not use a folder whose name includes blanks (for example "Program Files (x86)")

Step 3. Select the compilers to install
To perform minimal installation, choose the option Basic Setup from the left pane of the window, then select the check button for all compilers listed in the right pane of the window. More experienced users can choose the option All Packages and select the additional compilers they need.

Step 4. Click on each package with the right mouse button, then click on the Mark for Installation item
The minimal installation, "Basic Setup", includes 7 compilers which will be listed in the upper pane of the window. Right-click each one (or just the ones you want to install) and click the option Mark for Installation displayed in the context menu that will appear. This will cause an arrow icon to appear next to all compilers selected for installation.
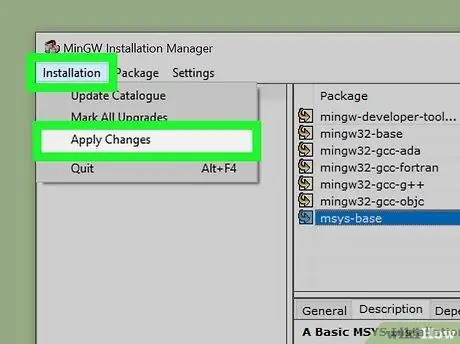
Step 5. Install the selected packages
It may take your computer several minutes to install all packages. Use the following instructions to install only the packages you have selected:
- Click on the menu Installation located in the upper left corner of the window;
- Click on the option Apply Changes;
- Click on the button Apply;
- Click on the button Close when the installation is complete.

Step 6. Add the path to the MinGW compiler installation folder inside the Windows system variables
Use the following instructions to complete this step:
- Type the environment command in the search bar of the "Start" menu;
- Click on the item Modify system-related environment variables appeared in the hit list;
- Click on the button Environment variables;
- Select the variable Path;
- Click on the button Edit placed under the upper pane of the window (called "User Variables");
- Click on the button New one;
- Type the code C: / MinGW / bin into the text field that appears - note that if you have installed the MinGW compiler in a directory other than the default, you will need to type the following code C: [install_path] bin;
- Click on the button in succession OK of both open windows, then on the button OK of the last window to close it.

Step 7. Open a "Command Prompt" window as a system administrator
To be able to perform this step, you will need to be logged in to Windows with a user account that is also a computer administrator. Follow these instructions to perform this step:
- Type the command cmd in the "Start" menu;
- Click on the icon Command Prompt appeared in the search results list, then select the option Run as administrator;
- Click on the button Yup to complete the request.
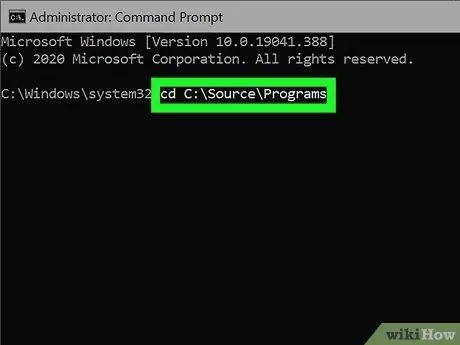
Step 8. Navigate to the folder where the file containing the source code to compile is stored
For example, if the program file to be compiled is called "helloworld.c" and is stored in the "C: / Sources / Program Files" folder, you will need to type the cd command C: / Sources / Program Files.
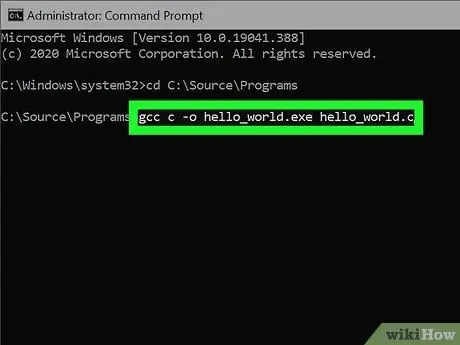
Step 9. Type the command gcc c -o [program_name].exe [program_name].c and press the Enter key
Replace the parameter "[program_name]" with the name of the file that contains the source code to compile. When compiling the program has finished without errors, the command prompt will reappear.
Any errors that will eventually be detected by the compiler will have to be corrected manually before the compilation can be completed

Step 10. Type the name of the compiled program to run it
If the file name is "hello_world.exe", type it into the "Command Prompt" to run the program.
If an error message similar to "Access denied" or "Permission denied" appears when compiling the code or while running the program, check the access permissions to the folder: you must make sure that your account has "read permissions" "and" write "for the folder where the program source code file is stored. If this solution doesn't fix the problem, try temporarily disabling your antivirus software
Advice
- Compiling the source code using the -g parameter will also generate the debug information by using the appropriate GDB program, which will make the debugging phase much more functional.
- Makefiles can be created to make it easier to compile very long programs.
- Be careful in trying to optimize your code for maximum performance while running the program, as you may end up with a large file or inaccurate and elegant code.
- To compile a program written in C ++ you will need to use the G ++ compiler in the same way you would use the GCC command. Remember that files written in C ++ have the extension ".cpp" instead of the extension ".c".