Have you always wondered how programs like Paint or Calculator are created? Well, learn how to create a simple Windows application using this step-by-step guide.
Steps

Step 1. Get a compiler
A compiler turns your raw source code (which you will write shortly) into an executable application. Obtain the DEV-CPP IDE software for the purposes of this tutorial. You can download it here.
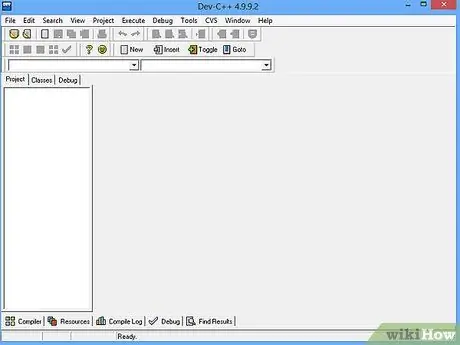
Step 2. Launch DEV-CPP once installed
You will be presented with a window with a text area where you will write your source code.

Step 3. Prepare to write a program to display text in a box
Before you start writing your source code, keep in mind that Win32 applications don't behave the same way as other programming languages, such as JAVA.
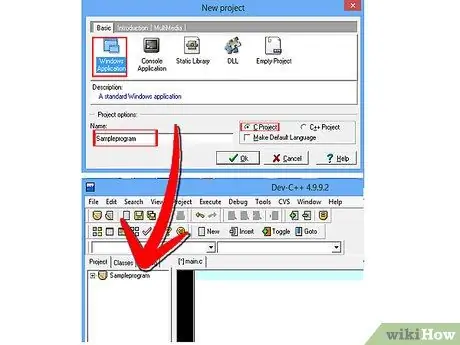
Step 4. On the DEV-CPP main screen, go to File -> New -> Project
You will be presented with another screen. Choose the icon where it says "Windows Application" and set the language as "C", not "C ++". In the text box where it says "Name", enter "ProgramExample". Now DEV-CPP will ask you where you want to save it. Save the file in any folder, but just make sure you remember it. Once this is done, you will be presented with a form on the source code screen. Press Ctrl + A and then Backspace. The reason we are doing this is that this way we can start over.
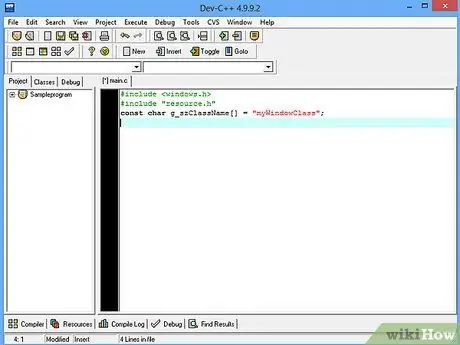
Step 5. At the beginning of your source code write "#include" (without quotes)
This includes the Windows library so you can create an application. Immediately below type: #include "resource.h" and then type: const char g_szClassName = "myWindowClass";
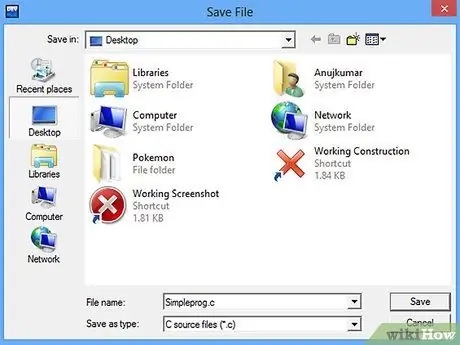
Step 6. Write a method to handle all messages and write another method where we will manage messages from resources
Don't worry if this confuses you. It will become clear later. For now, save your source code as ProgramExample.c. We will leave it as it is for the moment.

Step 7. Create a "Resource Script"
It is a piece of source code that defines all of your controls (for example: text boxes, buttons, etc.). You'll embed the resource script into your schedule and voila! You will have a schedule. Writing a resource script isn't difficult, but it takes time if you don't have a visual editor. This is because you will need to estimate the exact X and Y coordinates of the controls on the screen. On the DEV-CPP main screen, go to File -> New -> Resource File. DEV-CPP will ask you "Add resource files to current project?" Click on "Yes". At the beginning of the resource script, write #include "resource.h", and also write #include This takes care of all checks.

Step 8. Create your first control:
a simple menu. Write:
IDR_ILMENU MENU BEGIN POPUP "& File" BEGIN MENUITEM "E & xit", ID_FILE_EXIT END END
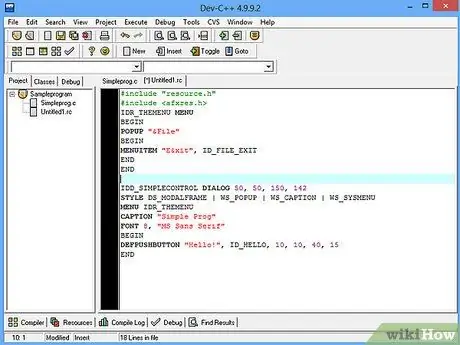
Step 9. Now let's move on to the buttons part
Your button will be placed inside a dialog, so we need to create the dialog first. To do this you need to write:
IDD_SIMPLECONTROL DIALOG 50, 50, 150, 142 STYLE DS_MODALFRAME | WS_POPUP | WS_CAPTION | WS_SYSMENU MENU IDR_ILMENU CAPTION "Example Program" FONT 8, "MS Sans Serif" BEGIN DEFPUSHBUTTON "Hello!", ID_CIAO, 10, 10, 40, 15 END

Step 10. Go to File -> New -> Source File
Add source file to current project? Yes. You will be presented with a blank screen. To assign values to our defined controls we need to give them numbers. It doesn't really matter what numbers you assign to your checks, but you should do it in a way that keeps them organized. For example, don't define a control by assigning a random number like 062491 or something else. So, write:
#define IDR_ILMENU 100 #define ID_FILE_EXIT 200 #define IDD_SIMPLECONTROL 300 #define ID_CIAO 400
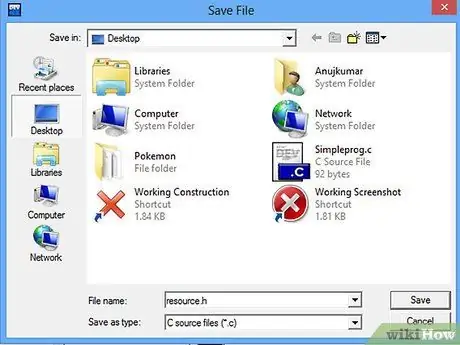
Step 11. Save this file as resource.h
Remember we created "#include" resource.h ""? Well, that's the reason we did it. We needed to assign values.
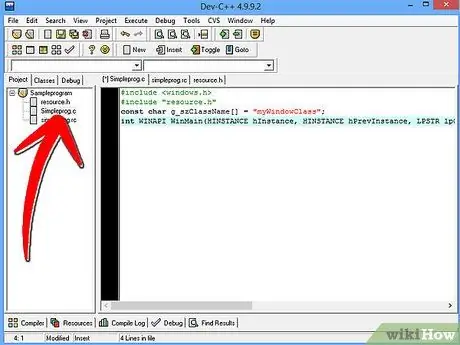
Step 12. Go back to the source, our ProgramExample.c or whatever you called it
Write:
int WINAPI WinMain (HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {return DialogBox (hInstance, MAKEINTRESOURCE (IDD_SIMPLECONTROL), NULL, SimpleProc);}

Step 13. Don't worry too much about the technical stuff here
Just understand that this part returns the dialog box to our message handling procedure called SimpleProc.
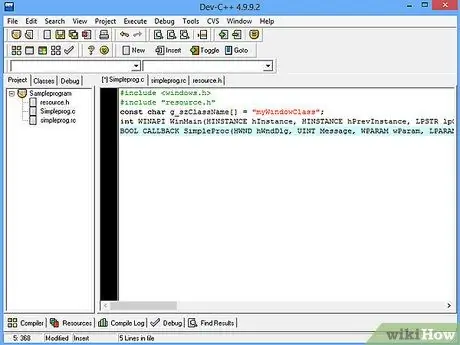
Step 14. Write:
BOOL CALLBACK SimpleProc (HWND hWndDlg, UINT Message, WPARAM wParam, LPARAM lParam) {switch (Message) {case WM_INITDIALOG: return TRUE; case WM_COMMAND: switch (LOWORD (wParam)) {case ID_CIAO: Message "NULL," HeyBox " "Hallo!", MB_OK) break; case ID_FILE_EXIT: EndDialog (hWndDlg, 0); break;} break; case WM_CLOSE: EndDialog (hWndDlg, 0); break; default: return FALSE;} return TRUE;}

Step 15. Make sure your SimpleProc comes before the int WINAPI WINMAIN
This is important if you want your program to work.
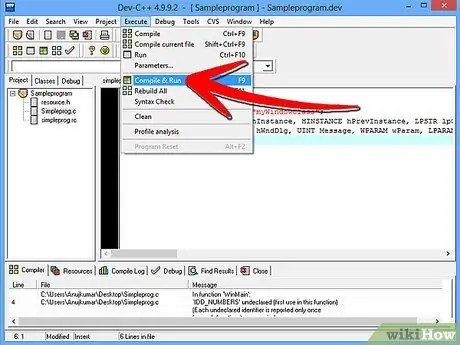
Step 16. Press F9 to compile and run your program
Advice
- If you are lost, there are many guides available on the internet.
- If you feel frustrated, take a break and then come back.
- This is a beginner's guide, so many parts are not explained. Although it is a beginner's guide, it is recommended that you have SOME experience in the world of programming (for example you understand logical operators like if-else etc.).