Are you stuck by the concept of "Function" in Visual Basic (VB)? If so, read this guide to learn about the constructs of functions according to the VB paradigms.
Steps

Step 1. What is a Function?
- Use a Function when you need to get a value to the calling code.
- The function itself has a type, and will call a value to the calling subroutine based on the code it contains.

Step 2. How to declare a Function?
- You can define a Function procedure only at the module level. This means that a function's declaration context must be a class, structure, module, or interface, and cannot be a source file, namespace, procedure, or block.
- A function is declared in the same way as a subroutine, with the only exception of using the word "Function" instead of "Sub".
- The Function procedure is public access by default. You can adjust their access level with access modifiers.
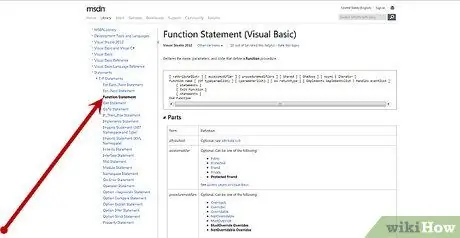
Step 3. How to call a Function?
- You call a Function procedure using the procedure name, followed by the argument in parentheses, in an expression.
- You can omit the brackets if you are not providing any arguments. However, your code will be more readable if you always include parentheses.
- You can also call a function using the Call statement, in which case the return value is ignored.
- To get a value, assign a value of the appropriate type to the function name, as if it were a variable.
Syntax
Declaration
[access modifier] [procedure modifier] [share] Function name [(Of parameter list type)] [(parameter list)] [As return type] [statements] [Exit function] [statements] End Function
Call
'Without Call Function_Name ()' With Call Call Function_Name ()
Example
Below you will find an example of a function that adds two numbers
Private Function Addizione (ByVal x As Integer, ByVal y As Integer) As Integer Dim Res as integer Res = x + y Addizione = Res End Function Private Sub Form_Carica () Dim a As Integer Dim b As Integer Dim c As Integer a = 32 b = 64 c = Addition (a, b) MsgBox ("The sum is:" & c) End Sub